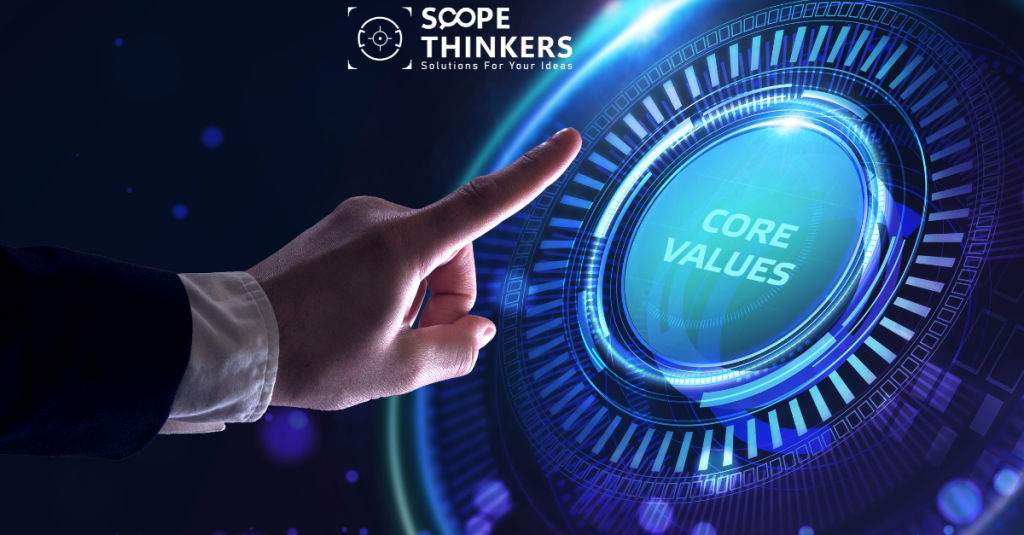
Testing is a crucial aspect of software development that ensures the reliability, functionality, and performance of applications. In the context of .NET Core APIs and Angular components, adopting effective testing strategies is essential to detect and prevent bugs, validate functionality, and maintain code quality. In this blog, we’ll explore comprehensive testing strategies for both .NET Core APIs and Angular components, covering unit testing, integration testing, and end-to-end testing techniques.
Unit Testing .NET Core APIs
Unit testing involves testing individual units or components of code in isolation to verify their correctness. For .NET Core APIs, unit tests typically focus on testing controllers, services, and data access logic. Here are some key strategies for unit testing .NET Core APIs:
- Use xUnit or NUnit: Choose a unit testing framework such as xUnit or NUnit to write and execute unit tests for your .NET Core APIs. These frameworks provide robust features for defining test cases, assertions, and test execution.
- Mock Dependencies: Use mocking frameworks like Moq or NSubstitute to mock external dependencies such as repositories, services, or external APIs. Mocking allows you to isolate the unit under test and control its behavior during testing.
- Test Controller Actions: Write unit tests to validate the behavior of controller actions, including input validation, HTTP responses, and error handling. Mock HTTP contexts and request/response objects to simulate HTTP requests and responses.
- Test Services and Business Logic: Test service classes and business logic components to ensure they produce the expected output given different input scenarios. Mock dependencies and use dependency injection to inject mock objects into the components under test.
- Test Data Access Logic: Write unit tests for data access logic such as repository methods or database queries. Mock database connections or use in-memory databases to isolate the data access layer from external dependencies.
Unit Testing Angular Components
Unit testing Angular components involves testing individual components in isolation to ensure they behave as expected. Here are some strategies for unit testing Angular components:
- Use Jasmine and Karma: Jasmine is a behavior-driven testing framework for JavaScript, and Karma is a test runner that executes JavaScript tests in various browsers. Use these tools to write and run unit tests for Angular components.
- Test Component Logic: Write tests to verify the logic and behavior of Angular components, including event handling, property binding, and DOM manipulation. Use Jasmine’s suite and spec functions to define test suites and individual test cases.
- Mock Dependencies: Mock external dependencies such as services, pipes, or child components using Jasmine’s spyOn function or Angular’s TestBed.configureTestingModule method. Mocking allows you to isolate the component under test and focus on its behavior.
- Test Input and Output Properties: Write tests to verify that input properties are correctly bound and output properties emit the expected values. Use Jasmine’s expect function to define assertions and validate component properties.
- Test Template Rendering: Write tests to ensure that the component’s template renders correctly and displays the expected content. Use Jasmine’s spyOn function to spy on DOM elements and verify their presence and properties.
Integration and End-to-End Testing
In addition to unit testing, integration testing and end-to-end testing are essential for validating the interactions between different components and ensuring the application functions as expected in a real-world environment. Here are some strategies for integration and end-to-end testing:
- Integration Testing .NET Core APIs: Write integration tests to validate the interactions between multiple components of the .NET Core API, such as controllers, services, and data access logic. Use tools like the ASP.NET Core TestHost to host the API in-memory and execute HTTP requests against it.
- End-to-End Testing Angular Applications: Use tools like Protractor or Cypress to write end-to-end tests for Angular applications. These tools simulate user interactions with the application and verify the behavior of the entire system, including frontend components, backend APIs, and database interactions.
- Test Scenarios and User Flows: Write integration and end-to-end tests to validate common user scenarios and user flows within the application. Test user authentication, form submissions, data retrieval, and other critical functionalities to ensure they work as expected.
- Automate Testing with CI/CD Pipelines: Integrate testing into your CI/CD pipelines to automate the execution of unit tests, integration tests, and end-to-end tests whenever changes are made to the codebase. Use tools like Azure DevOps or GitHub Actions to set up automated testing workflows.
- Monitor Test Coverage and Performance: Keep track of test coverage metrics to ensure sufficient test coverage across different parts of the application. Monitor test execution times and performance metrics to identify bottlenecks and optimize testing processes.
Conclusion
Comprehensive testing is essential for ensuring the reliability, functionality, and performance of .NET Core APIs and Angular components. By adopting effective testing strategies, including unit testing, integration testing, and end-to-end testing, you can detect and prevent bugs early in the development lifecycle, validate functionality across different layers of the application, and deliver high-quality software that meets user expectations. Incorporate testing into your development workflow, leverage automated testing tools and frameworks, and continuously monitor test coverage and performance to ensure the success of your .NET Core and Angular projects.